StateGroups – finally found a use for them
State groups? Ever looked at them and though they look really useful but then can’t find a good use for them!
Well last year (yes its taken a while to finish of this post – hopefully its worth it), while developing some mobile apps I came across a great use for them. At the time their were also very few examples of using stategroups on-line and none in a practical setting.
So here goes, lets see how they’re done and where would be good to use them.
- The problem
- That give is 2 different groups of states
- Soloution
Mobile apps can have 1 of 3 DPI settings and this will change how your app looks. (320 DPI, 240 DPI and 120 DPI).
So we can think of each of these DPI’s as a state.
Then we can have a view and like most views you will use states. For example to have “Edit mode on” and “Edit mode off”.
Group 1 is the DPI setting.
Group 2 is the view mode.
I always like to see working examples of something, bit like a picture & a 1000 words.
So below is a test app to change the values of and of course it has source code to view.
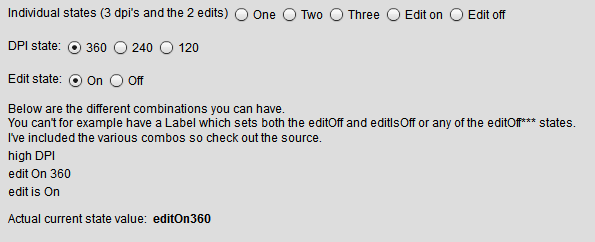
I’ve also included below what I consider to be the important bits of the code to understand.
Check out the source code of the app for more detail.
These are the various combinations of states you can use together, and the list of the actual states.
For example you can’t set text.one and text.lowDPI on the same component.
Hopefully this will give you a bit of insight into how and where to use stateGroups. Very useful tool under specific circumstances.
[ad name=”ad-1″]